gem sources -a http://gems.github.com
gem install jpignata-bossman
Programming
Programming is all about solving problems and fun.
Thursday, September 4, 2008
Yahoo! BOSS for Ruby
Yahoo! launched BOSS. It is a service that allows you to search the entire Yahoo! Search index. It is really cool. Meanwhile, Jay Pignata has developed a ruby library, BOSSMan, for Rubyists to mess around with Yahoo! search web service. BOSSMan lives on Github. If you wanna try just:
Friday, August 15, 2008
Prawn generates PDF
You can use Prawn to generate PDF. Prawn is a ruby library that make PDF creation possible in Ruby. more
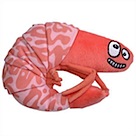
Thursday, August 14, 2008
Ruby to JavaScript
If you are a fan of Ruby and you want to write JavaScript code, you don't need to learn JavaScript. You can write JavaScript code by using RED. RED is a ruby library that will convert Ruby code to JavaScript code. Check this out!
Thursday, July 3, 2008
Passing arguments from method to method in JavaScript
For example, you have one function greeting() that accepts two string arguments only. And you have another function callAnotherFunction() that accepts only one argument which is a function. In order to pass arguments from method to method, JavaScript provide ideal apply() and call() to deal with this scenario.
These example might not make sense to some people but it very useful when you want to pass arguments from method to method.
Hope this help.
function greeting(name, str) {
alert(str + ", " + name);
}
function callAnotherFunction(method) {
method.apply(null, arguments); // arguments refer to ["John", "Good morning"]
alert(arguments.length); // arguments refer to method
}
callAnotherFunction(function() {greeting("John", "Good morning")});
The significant point in this example is arguments
in callAnotherFunction(method).
It means differently in the same function. In method.apply(null, arguments),
it refers to the arguments that passed from method. And in alert(arguments.length),
it refers to method itself, not its arguments.These example might not make sense to some people but it very useful when you want to pass arguments from method to method.
Hope this help.
Wednesday, July 2, 2008
Good practice in array deletion in JavaScript
It is really piss me off when I spent a few hours trying to find the failure in my JavaScript code.
Eventually, I found out what the problem was. I did not delete my array correctly.
This for loop never delete all the elements in your array. When i = 0, arr[0] (a) is deleted and when i = 1, arr[1] (c) is deleted and then exit the loop cause i eqauls arr.length equals 2.
To deal with this problem, you should assing arr.length to one variable so when you delete the array it does not effect you loop.
Hope this help.
Eventually, I found out what the problem was. I did not delete my array correctly.
var arr = [1, 2, 3, 4];
for (var i=0; i<arr.length; i++)
arr.splice(i, 1);
This for loop never delete all the elements in your array. When i = 0, arr[0] (a) is deleted and when i = 1, arr[1] (c) is deleted and then exit the loop cause i eqauls arr.length equals 2.
To deal with this problem, you should assing arr.length to one variable so when you delete the array it does not effect you loop.
var arr = [1, 2, 3, 4];
for (i=0, len=arr.length; i<len; i++)
arr.splice(0,1);
Hope this help.
Tuesday, June 10, 2008
What happen in DOM?
I have discovered the unexpected behavior of DOM when I was writing some JavaScript code. The example will illustrate the problem
// Create one container and add to two children to it
var container1 = document.createElement("DIV"); container1.appendChild(document.createElement("DIV"));
container1.appendChild(document.createElement("DIV"));
//Now I want to the two children in container to document.body
//Apparently, the code in the comment below should work
//document.body.appendChild(container1.childNodes[0]);
//document.body.appendChild(container1.childNodes[1]);
//Unfortunately, it does not work. When we appended the first child in container1 to
//document.body the browser automatically deleted that child from container. So,
//after the insertion container1 has only one child not two. The code should be like
//the below code:
document.body.appendChild(container1.childNodes[0]);
document.body.appendChild(container1.childNodes[0]);
Hope this help.
// Create one container and add to two children to it
var container1 = document.createElement("DIV"); container1.appendChild(document.createElement("DIV"));
container1.appendChild(document.createElement("DIV"));
//Now I want to the two children in container to document.body
//Apparently, the code in the comment below should work
//document.body.appendChild(container1.childNodes[0]);
//document.body.appendChild(container1.childNodes[1]);
//Unfortunately, it does not work. When we appended the first child in container1 to
//document.body the browser automatically deleted that child from container. So,
//after the insertion container1 has only one child not two. The code should be like
//the below code:
document.body.appendChild(container1.childNodes[0]);
document.body.appendChild(container1.childNodes[0]);
Hope this help.
Tuesday, March 18, 2008
Fixed position in IE
IE does not support position: fixed like Firefox does. But we still have way to make it possible.
/* set background url to whatever you want and then make it fixed position.*/
body{
background:url(foo1) fixed;
}
/* this is the div that you want to be fix */
#my_div{
position:fixed;
_position:absolute;
top:0;
_top:expression(eval(document.body.scrollTop));
left:0;
margin:0;
padding:0;
background:lime;
}
Actually, I got this stuff from http://annevankesteren.nl/test/examples/ie/position-fixed.html
Hope this help.
/* set background url to whatever you want and then make it fixed position.*/
body{
background:url(foo1) fixed;
}
/* this is the div that you want to be fix */
#my_div{
position:fixed;
_position:absolute;
top:0;
_top:expression(eval(document.body.scrollTop));
left:0;
margin:0;
padding:0;
background:lime;
}
Actually, I got this stuff from http://annevankesteren.nl/test/examples/ie/position-fixed.html
Hope this help.
Problems RMagick and RubyGems
It will have some problems if you use try to use RMagick with RubyGem > 0.9.For instance, if you use drawing primitive methods, simply you will see (no primitives defined):Magick::Draw error message. To deal with this error, it is not very difficult. You just go to your require statement and change form require 'RMagick' to require 'RMagic.rb'. See the difference.
Hope this help.
Hope this help.
Friday, March 7, 2008
RMagick unable to read font n021004l.pfb solution
During my development process, I have encountered one problem with RMagick. RMagick was unable to read font n021004l.pfb. Let assume that you have installed RMagick in C:\Program Files\ImageMagick-6.3.0-Q8. Then go to folder config in your RMagick folder and open a file name type.xml and comment
Hope this help.
<!--<include file="type-ghostscript.xml" />-->
Hope this help.
Subscribe to:
Posts (Atom)